|
Host-Based Motion
|
|
Basic
Motion |
NOTE: The terms "Control object" and "Controller"
designate the same thing: a Control object.
To perform a simple motion using the XMP controller requires
as few as eight basic steps:
- Create a Control object
- Initialize the Control object
- Create an Axis Object
- Create a Motion Object
- Append an Axis to a Motion object's list (of axes)
- Start the Motion Check the Status of the Motion
- Delete the Objects
In more detail,
- Create a Control Object: Use mpiControlCreate(...)
to create a Control object. You should only create one
and only one Control object per board per process. The
Control object controls all of the operating system resources
for the actual board. You must create a Control object
before creating any other objects.
- Initialize the Control Object: Use mpiControlInit(...)
to initialize a Control object. mpiControlInit(...) establishes
contact with the device driver, and maps the XMP's memory
space to the application's memory space. An application
can call mpiControlInit(...) more than once, but it isn't
necessary.
- Create an Axis Object: Use mpiAxisCreate(...)
to create an Axis object, by passing a Control object
handle and Axis number to it.
- Create a Motion Object: Use mpiMotionCreate(...)
to create a Motion object, by passing a Control object
handle and a Motion Supervisor (number) to it. Each motion
object maintains a list of axes, which defines a coordinate
system (specified by the number and order of the axes).
- Add an Axis to a Motion object's list (of axes):
Use mpiMotionAxisAppend(...) to associate an Axis with
a Motion. The association is done by appending the Axis
to a Motion object's list of axes. Note that the Axis
must be located on the same controller (board) as the
Motion object.
- Start the Motion: Use mpiMotionStart(...) to
start a Motion.
- Check the Status of the Motion: Use mpiMotionStatus(...)
to determine if the current motion has completed yet.
- Delete the Objects: Use mpiObjectDelete(...)
methods to delete the objects. We recommend deleting objects
in the reverse order of creation. For this example, you
would perform the object deletions in the order: Motion
first, then Axis, then Control. Note that for any application,
the Control object must be deleted last.
|
|
|
Controller-Based Motion
|

|
Motion Command
|
The XMP controller's firmware uses
a state machine for controller-based motion. The motion state
machine has three states (Moving, Idle, Error). Transitions
between states occur after a command (Start, Resume, Modify,
Stop, E-Stop, Abort, Reset) or after an event (Done, Amp_Fault,
Limits). |
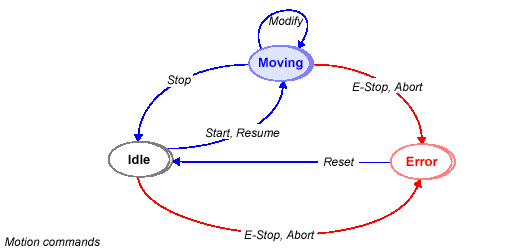 |
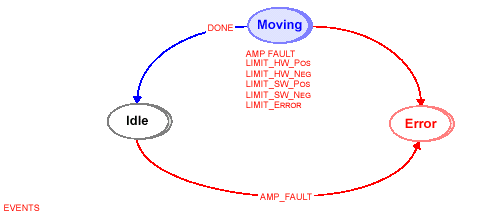 |
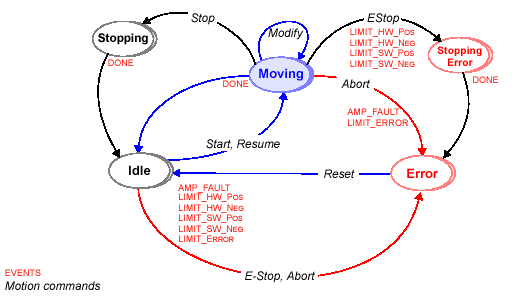 |
MPIEvents are generated by the XMP controller.
MPIEvents (axis events) cause MPIActions to be generated. The
MPIActions in the table are the default values and can be changed.
Valid MPIActions for events are None, Stop, EStop, Abort. |
MPIEventType |
MPIAction (Default) |
Event
Trigger |
AMP_FAULT |
ABORT |
Polarity
(I/O bit Hi/Lo) |
HOME |
STOP |
Polarity (I/O bit Hi/Lo) |
LIMIT_HW_POS |
ESTOP |
Polarity (I/O bit Hi/Lo) |
LIMIT_HW_NEG |
ESTOP |
Polarity (I/O bit Hi/Lo) |
LIMIT_SW_POS |
ESTOP |
Position |
LIMIT_SW_NEG |
ESTOP |
Position |
LIMIT_ERROR |
ABORT |
Error [= absolute value (command
position - actual position)] |
|
MPIActions can come from the host or the firmware. For safety,
there are 4 guaranteed restrictions on who can generate certain
MPIActions:
|
MPIAction |
Originating from Host |
Originating from XMP Firmware |
Start
Resume
Reset
Stop |
mpiMotionStart(...) mpiMotionResume(...)
mpiMotionReset(...)
mpiMotionStop(...) |
Never 1
Never 1
Never 1
Event |
E_Stop
Abort |
mpiMotionEStop(...) mpiMotionAbort(...)
|
Event
Event |
Done |
Never 2
|
If Settled and In_Position |
1 = Start/Resume/Reset can never
be issued from the firmware; Start/Resume/Reset can only be
issued from the host.
2 = Done can never be issued
from the host; Done can only be issued from the firmware.
|
|
|
Sequence
|
|
A Sequence consists of a linked list of Commands. Execution
of a Sequence can be started, stopped, or its status queried.
Execution of a Sequence begins with the first Command in the
sequence and proceeds sequentially, unless otherwise commanded
(e.g., a branch command). Execution of a Sequence ends when
the last Command in the sequence is executed, or when the
execution is stopped by the host.
An XMP can be controlled directly by the host or controlled
by commands that the host downloads (for independent execution
by the XMP firmware). Downloaded commands are managed by a
firmware object known as a Program Sequencer (PS).
|
|
For the XMP, there are currently 16 Program Sequencers and
a single command buffer that holds 256 commands. Each Sequence
is created with a maximum command count, but the total command
count for all Program Sequencers cannot exceed 256 commands.
|
|
The XMP commands can be thought of as a machine language for
a virtual RISC processor (Reduced Instruction Set Computer)
implemented by the XMP firmware. Similarly, a Program Sequencer
can be thought of as an operating system thread, with the PS
command buffer considered as a page of virtual memory for the
thread. Each PS maintains the location within its command buffer
of the next command to be executed, i.e., a program counter.
The XMP firmware time-slices the PS "threads" by executing a
command in every active PS, on every firmware cycle. When the
next command to be executed is not located in the PS command
buffer, a host interrupt is generated (i.e., a page fault occurs).
The host is then expected to fill the PS command buffer with
the next batch of commands. This method allows command sequences
to be of any length. |
|
The Motion Programming Interface (MPI) provides a layer of
abstraction on top of the XMP Program Sequencer. The MPI Sequence
object maintains a list of MPI Command objects. Commands exist
to perform motion, compute/load/store data, branch-on/wait-for
conditions (arithmetic, logical, I/O bits, events), delay,
generate user-defined events, etc. There are a variety of
command types, each with type-specific command parameters.
The high-level language defined by these commands makes use
of MPI objects, and allows for simple expressions with operands
(that can be specified by value or by reference). A command
can have a text label so that the command itself can be the
target of a branch command.
When you create a Sequence, you specify the desired XMP PS
command buffer size. Specifying a PS command buffer size of
-1 will cause all remaining command buffer space to be allocated.
After creating a Sequence, you typically create and append
Command objects to the Sequence, and a Sequence can have any
number of Command objects appended to it. When the Sequence
is started, the Commands are "compiled" into XMP commands
and loaded into the PS command buffer for execution.
When the XMP firmware does not find the next command to be
executed in the command buffer, the firmware generates a host
interrupt. This interrupt is handled by the MPI EventMgr,
which then calls the Sequence to load the next batch of commands
down to the Program Sequencer; your application does not have
to do anything.
|
|
|
|